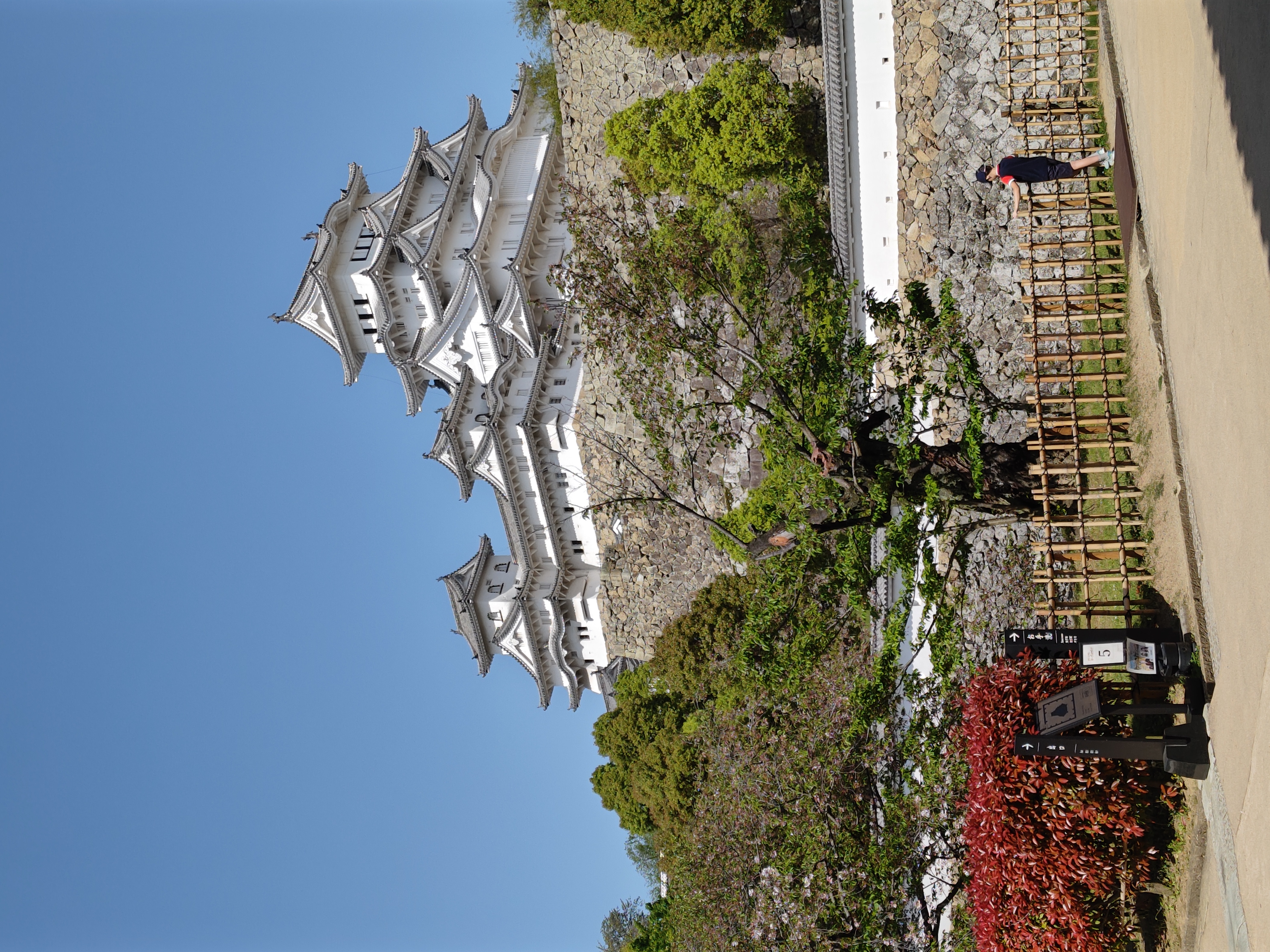
Osiro.JPG
Node.jsとViteプロジェクト作成
資料
基本コマンド
node -v
cd ~/Desktop
mkdir vite
cd vite
npm create vite@latest :新しいReactプロジェクトの作成
cd <project-name> :依存関係のインストール
npm install
npm run dev
1、 index.html作成
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>My Vite Project</title>
</head>
<body>
<h1>Hello, Vite!</h1>
<p>This is my custom page.</p>
</body>
</html>
2 , main.jsまたはmain.tsの編集
JavaScript/TypeScriptのコードを追加します。
// src/main.js
import './style.css';
document.querySelector('#app').innerHTML = `
<h1>Hello Vite! </h1>
import React from 'react';
import ReactDOM from 'react-dom/client';
import App from './App.jsx';
import './index.css';
ReactDOM.createRoot(document.getElementById('root')).render(
<React.StrictMode>
<App />
</React.StrictMode>
);
a href="https://vitejs.dev/guide/features.html" target="_blank">Documentation </a>
`;
3, 変更内容の反映
npm run devを実行中であれば、保存するたびにブラウザが自動的に更新され、変更内容が反映されます。
この手順を通じて、Node.jsおよびViteをインストールし、独自のWebページを表示することができます。
プロジェクト構造の確認と編集
srcディレクトリ内のファイルを以下のように設定します。
src/main.jsx
import React from 'react';
import ReactDOM from 'react-dom/client';
import App from './App.jsx';
import './index.css';
ReactDOM.createRoot(document.getElementById('root')).render(
<React.StrictMode>
<App />
</React.StrictMode>
);
次に、App.jsxを編集します。このファイルに表示したいコンテンツを追加します。
src/App.jsx
import React from 'react';
const App = () => {
return (
<div>
<h1>Hello Vite! </h1>
<a href="https://vitejs.dev/guide/features.html" target="_blank">Documentation </a>
</div>
);
};
export default App;
必要に応じてスタイルを設定します。ここでは、基本的なスタイルの例を示します。
src/index.css
body {
font-family: Arial, sans-serif;
margin: 0;
padding: 0;
background-color: #f0f0f0;
}
h1 {
color: #333;
}
a {
color: #007bff;
text-decoration: none;
}
a:hover {
text-decoration: underline;
}
ローカルサーバーの起動
以下のコマンドを実行して、開発サーバーを起動します。
npm run dev
src/App.jsx コード にheader やnav を追加
更新された src/App.jsx
以下のように各セクションのコンテンツを追加します。リンクをクリックすると対応するセクションにスクロールするようにします。
import React from 'react';
const App = () => {
return (
<div>
<header>
<h1>My Website</h1>
<nav>
<ul>
<li><a href="#home">Home</a></li>
<li><a href="#about">About</a></li>
<li><a href="#services">Services</a></li>
<li><a href="#contact">Contact</a></li>
</ul>
</nav>
</header>
<main>
<section id="home">
<h2>Home</h2>
<p>Welcome to the homepage!</p>
</section>
<section id="about">
<h2>About</h2>
<p>This is the about section.</p>
</section>
<section id="services">
<h2>Services</h2>
<p>Here are the services we offer.</p>
</section>
<section id="contact">
<h2>Contact</h2>
<p>Get in touch with us!</p>
</section>
<h1>Hello Vite!</h1>
<a href="https://vitejs.dev/guide/features.html" target="_blank">Documentation</a>
</main>
</div>
);
};
export default App;
スタイルの追加(オプション)
src/index.cssに各セクションのスタイルを追加します
body {
font-family: Arial, sans-serif;
margin: 0;
padding: 0;
background-color: #f0f0f0; // 背景色を薄いグレー 白
}
header {
background-color: #282c34; //重い青
padding: 20px;
color: white;
}
header h1 {
margin: 0;
display: inline-block;
}
nav ul {
list-style-type: none;
padding: 0;
}
nav ul li {
display: inline;
margin-right: 20px;
}
nav ul li a {
color: white;
text-decoration: none;
}
nav ul li a:hover {
text-decoration: underline;
}
main {
padding: 20px;
}
section {
padding: 20px 0;
}
section:nth-child(odd) {
background-color: #fff;
}
section:nth-child(even) {
background-color: #f9f9f9;
}
a {
color: #007bff;
text-decoration: none;
}
a:hover {
text-decoration: underline;
}
header内にリンクを追加し、それらのリンク先のファイルを作成する方法
1. header内にリンクを追加
まず、header内にリンクを追加します。リンクはReact RouterのLinkコンポーネントを使用します。
更新されたsrc/App.jsx
import React from 'react';
import { BrowserRouter as Router, Route, Routes, Link } from 'react-router-dom';
import Home from './pages/Home';
import About from './pages/About';
import Services from './pages/Services';
import Contact from './pages/Contact';
const App = () => {
return (
<Router>
<div>
<header>
<h1>My Website</h1>
<nav>
<ul>
<li><Link to="/">Home</Link></li>
<li><Link to="/about">About</Link></li>
<li><Link to="/services">Services</Link></li>
<li><Link to="/contact">Contact</Link></li>
</ul>
</nav>
</header>
<main>
<Routes>
<Route path="/" element={<Home />} />
<Route path="/about" element={<About />} />
<Route path="/services" element={<Services />} />
<Route path="/contact" element={<Contact />} />
</Routes>
</main>
</div>
</Router>
);
};
export default App;
2, 各リンク先のコンポーネントを作成
次に、各リンク先のコンポーネントを作成します。
src/pages/Home.jsx
import React from 'react';
const Home = () => {
return (
<section>
<h2>Home
<p>Welcome to the homepage!
</section>
);
};
export default Home;
src/pages/About.jsx
import React from 'react';
const About = () => {
return (
<section>
<h2>About</h2>
<p>This is the about section.</p>
</section>
);
};
export default About;
src/pages/Services.jsx
import React from 'react';
const Services = () => {
return (
<section>
<h2>Services</h2>
<p>Here are the services we offer.</p>
</section>
);
};
export default Services;
src/pages/Contact.jsx
import React from 'react';
const Contact = () => {
return (
<section>
<h2>Contact</h2>
<p>Get in touch with us!</p>
</section>
);
};
export default Contact;
3. React Routerをインストールしてルーティングを設定
React Routerをインストールして、ルーティングを設定します。
React Routerのインストール
npm install react-router-dom
画像ファイルをReactアプリケーションのHome画面に反映
osiro.JPGファイルをプロジェクトのsrcディレクトリ内に追加します。例えば、
src/assetsフォルダを作成し、その中に画像ファイルを置くことが一般的です。
vite-project/
├── src/
│ ├── assets/
│ │ └── osiro.JPG
│ ├── App.jsx
│ ├── main.jsx
│ └── pages/
│ └── Home.jsx
Homeコンポーネントに画像を表示するコードを追加します。
src/pages/Home.jsx
import React from 'react';
import osiroImage from '../assets/osiro.JPG';
const Home = () => {
return (
<section>
<h2>Home</h2>
<p>Welcome to the homepage!</p>
<img src={osiroImage} alt="Oshiro" />
</section>
);
};
export default Home;
App.jsxにHomeコンポーネントが正しく設定されているか確認してください。以下はその確認です。
src/App.jsx
import React from 'react';
import { BrowserRouter as Router, Route, Routes, Link } from 'react-router-dom';
import Home from './pages/Home';
import About from './pages/About';
import Services from './pages/Services';
import Contact from './pages/Contact';
const App = () => {
return (
<Router>
<div>
<header>
<h1>My Website</h1>
<nav>
<ul>
<li><Link to="/">Home</Link></li>
<li><Link to="/about">About</Link></li>
<li><Link to="/services">Services</Link></li>
<li><Link to="/contact">Contact</Link></li>
</ul>
</nav>
</header>
<main>
<Routes>
<Route path="/" element={<Home />} />
<Route path="/about" element={<About />} />
<Route path="/services" element={<Services />} />
<Route path="/contact" element={<Contact />} />
</Routes>
</main>
</div>
</Router>
);
};
export default App;
開発サーバーを起動してnpm run dev
、変更を確認します。
Viteで画像ファイル(.JPG)をインポートする際にエラーが発生する場合
Viteの設定ファイルにアセットインクルードを追加
まず、プロジェクトのルートにある vite.config.js ファイルを開き、以下のようにアセットとしてJPEGファイルを含める設定を追加します。
vite.config.js
import { defineConfig } from 'vite';
import react from '@vitejs/plugin-react';
export default defineConfig({
plugins: [react()],
assetsInclude: ['**/*.JPG']
});
Reactコンポーネントで画像ファイルをインポートして使用します。
src/pages/Home.jsx
import React from 'react';
import osiroImage from '../assets/osiro.JPG';
const Home = () => {
return (
<section>
<h2>Home</h2>
<p>Welcome to the homepage!</p>
<img src={osiroImage} alt="Oshiro" />
</section>
);
};
export default Home;
Viteの設定ファイルを変更したので、開発サーバーを再起動するnpm run dev
必要があります。